Implementation Design
This is a unordered list of implementation design decisions. Each topic tries to follow this structure:
- Problem statement
- Proposed Solution
- Alternatives and Discussion
Coverage Analysis Mechanism
Coverage information has to be collected at runtime. For this purpose JaCoCo creates instrumented versions of the original class definitions. The instrumentation process happens on-the-fly during class loading using so called Java agents.
There are several different approaches to collect coverage information. For each approach different implementation techniques are known. The following diagram gives an overview with the techniques used by JaCoCo highlighted:
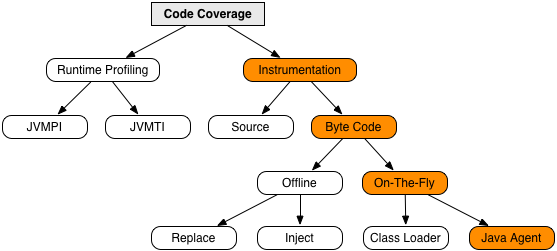
Byte code instrumentation is very fast, can be implemented in pure Java and works with every Java VM. On-the-fly instrumentation with the Java agent hook can be added to the JVM without any modification of the target application.
The Java agent hook requires at least 1.5 JVMs. Class files compiled with debug information (line numbers) allow for source code highlighting. Unluckily some Java language constructs get compiled to byte code that produces unexpected highlighting results, especially in case of implicitly generated code like default constructors or control structures for finally statements.
Coverage Agent Isolation
The Java agent is loaded by the application class loader. Therefore the classes of the agent live in the same name space like the application classes which can result in clashes especially with the third party library ASM. The JoCoCo build therefore moves all agent classes into a unique package.
The JaCoCo build renames all classes contained in the
jacocoagent.jar
into classes with a
org.jacoco.agent.rt_<randomid>
prefix, including the
required ASM library classes. The identifier is created from a random number.
As the agent does not provide any API, no one should be affected by this
renaming. This trick also allows that JaCoCo tests can be verified with
JaCoCo.
Minimal Java Version
JaCoCo requires Java 1.5.
The Java agent mechanism used for on-the-fly instrumentation became available with Java 1.5 VMs. Coding and testing with Java 1.5 language level is more efficient, less error-prone – and more fun than with older versions. JaCoCo will still allow to run against Java code compiled for these.
Byte Code Manipulation
Instrumentation requires mechanisms to modify and generate Java byte code. JaCoCo uses the ASM library for this purpose internally.
Implementing the Java byte code specification would be an extensive and error-prone task. Therefore an existing library should be used. The ASM library is lightweight, easy to use and very efficient in terms of memory and CPU usage. It is actively maintained and includes a huge regression test suite. Its simplified BSD license is approved by the Eclipse Foundation for usage with EPL products.
Java Class Identity
Each class loaded at runtime needs a unique identity to associate coverage data with. JaCoCo creates such identities by a CRC64 hash code of the raw class definition.
In multi-classloader environments the plain name of a class does not unambiguously identify a class. For example OSGi allows to use different versions of the same class to be loaded within the same VM. In complex deployment scenarios the actual version of the test target might be different from current development version. A code coverage report should guarantee that the presented figures are extracted from a valid test target. A hash code of the class definitions allows to differentiate between classes and versions of classes. The CRC64 hash computation is simple and fast resulting in a small 64 bit identifier.
The same class definition might be loaded by class loaders which will result in different classes for the Java runtime system. For coverage analysis this distinction should be irrelevant. Class definitions might be altered by other instrumentation based technologies (e.g. AspectJ). In this case the hash code will change and identity gets lost. On the other hand code coverage analysis based on classes that have been somehow altered will produce unexpected results. The CRC64 code might produce so called collisions, i.e. creating the same hash code for two different classes. Although CRC64 is not cryptographically strong and collision examples can be easily computed, for regular class files the collision probability is very low.
Coverage Runtime Dependency
Instrumented code typically gets a dependency to a coverage runtime which is responsible for collecting and storing execution data. JaCoCo uses JRE types only in generated instrumentation code.
Making a runtime library available to all instrumented classes can be a
painful or impossible task in frameworks that use their own class loading
mechanisms. Since Java 1.6 java.lang.instrument.Instrumentation
has an API to extends the bootsstrap loader. As our minimum target is Java 1.5
JaCoCo decouples the instrumented classes and the coverage runtime through
official JRE API types only. The instrumented classes communicate through the
Object.equals(Object)
method with the runtime. A instrumented
class can retrieve its probe array instance with the following code. Note
that only JRE APIs are used:
Object access = ... // Retrieve instance Object[] args = new Object[3]; args[0] = Long.valueOf(8060044182221863588); // class id args[1] = "com/example/MyClass"; // class name args[2] = Integer.valueOf(24); // probe count access.equals(args); boolean[] probes = (boolean[]) args[0];
The most tricky part takes place in line 1 and is not shown in the snippet
above. The object instance providing access to the coverage runtime through
its equals()
method has to be obtained. Different approaches have
been implemented and tested so far:
SystemPropertiesRuntime
: This approach stores the object instance under a system property. This solution breaks the contract that system properties must only containjava.lang.String
values and therefore causes trouble in applications that rely on this definition (e.g. Ant).LoggerRuntime
: Here we use a sharedjava.util.logging.Logger
and communicate through the logging parameter array instead of aequals()
method. The coverage runtime registers a customHandler
to receive the parameter array. This approach might break environments that install their own log managers (e.g. Glassfish).ModifiedSystemClassRuntime
: This approach adds a public static field to an existing JRE class through instrumentation. Unlike the other methods above this is only possible for environments where a Java agent is active.InjectedClassRuntime
: This approach defines a new class usingjava.lang.invoke.MethodHandles.Lookup.defineClass
introduced in Java 9.
Starting from version 0.8.3 JaCoCo Java agent implementation uses the
InjectedClassRuntime
to define new class in bootstrap class
loader when running on JRE 9 and higher, otherwise uses
ModifiedSystemClassRuntime
to add field to an existing JRE class.
Starting from version 0.8.0 field is added to the class
java.lang.UnknownError
, versions 0.5.0 - 0.7.9 were adding field
to the class java.util.UUID
, having bigger chance of conflict
with other agents.
Memory Usage
Coverage analysis for huge projects with several thousand classes or hundred thousand lines of code should be possible. To allow this with reasonable memory usage the coverage analysis is based on streaming patterns and "depth first" traversals.
The complete data tree of a huge coverage report is too big to fit into a reasonable heap memory configuration. Therefore the coverage analysis and report generation is implemented as "depth first" traversals. Which means that at any point in time only the following data has to be held in working memory:
- A single class which is currently processed.
- The summary information of all parents of this class (package, groups).
Java Element Identifiers
The Java language and the Java VM use different String representation formats
for Java elements. For example while a type reference in Java reads like
java.lang.Object
, the VM references the same type as
Ljava/lang/Object;
. The JaCoCo API is based on VM identifiers only.
Using VM identifiers directly does not cause any transformation overhead at runtime. There are several programming languages based on the Java VM that might use different notations. Specific transformations should therefore only happen at the user interface level, for example during report generation.
Modularization of the JaCoCo implementation
JaCoCo is implemented in several modules providing different functionality. These modules are provided as OSGi bundles with proper manifest files. But there are no dependencies on OSGi itself.
Using OSGi bundles allows well defined dependencies at development time and at runtime in OSGi containers. As there are no dependencies on OSGi, the bundles can also be used like regular JAR files.